15 Sep 2013 18:46:26
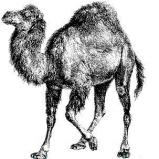
Perl Script
Данный скрипт удаляет мусор из css файла по указанным селекторам.Bash part:
#!/bin/bash if [ -f $1 ] then selectors=$(cat `pwd`'/selectors') content=`cat $1` IFS=$(echo -en "\n\b") for i in $selectors do content="$(echo "$content" | perl `pwd`/regexp.pl "$i" | more)" done #content="$(echo "$content" | perl `pwd`/formater.pl | more)" echo "$content" > $1 else echo "error, there isn't exist $1 file" fi
Perl part:
#!/usr/bin/perl # Created by Pavel Ruban. # Contacts: http://pavelruban.org BEGIN { $selector = $ARGV[0] } $content = <STDIN>; # Declare the subroutines sub trim($); ## Function block. # Delete css block with single matched selector or delete selector's part mattched. sub complex_selector_exclude { my (@selectors) = @_; foreach my $selector (@selectors) { $selector =~ s/(\\ |\\s)/\\s+/g; BEGIN {undef $/;} $content =~ s/^\s*($selector)\s*{[^{]*?}//gsm; $content =~ s/^([^{]*),\s*(?:$selector)\s*([^{]*{)/$1 $2/gsm; $content =~ s/^([^{]*)\s*(?:$selector)\s*,([^{]*{)/$1 $2/gsm; } return $content; } # Delete css block with single matched selector or delete selector's part mattched. sub simple_selector_exclude { my ($selector) = @_; $selector =~ s/(\\ |\\s)/\\s+/g; BEGIN {undef $/;} $content =~ s/^\s*($selector)\s*{[^{]*?}//gsm; $content =~ s/^([^{]*),\s*(?:$selector)\s*([^{]*{)/$1 $2/gsm; $content =~ s/^([^{]*)\s*(?:$selector)\s*,([^{]*{)/$1 $2/gsm; return $content; } ## Main logic block. if ($selector =~ /,/) { @selectors = split(/,/, $selector); foreach my $selector (@selectors) { $trimmedSelector = trim($selector); if ($trimmedSelector) { $trimmedSelector = quotemeta($trimmedSelector); push(@checked_selectors, $trimmedSelector); } } if (!@checked_selectors) { # Output script result. print $content; exit; } } else { $checked_selector = trim($selector); if (!$checked_selector) { # Output script result. print $content; exit; } $checked_selector = quotemeta($checked_selector); } if (@checked_selectors) { $content = complex_selector_exclude(@checked_selectors); } else { $content = simple_selector_exclude($checked_selector); } # Output script result. print $content; # Perl trim function to remove whitespace from the start and end of the string sub trim($) { my $string = shift; $string =~ s/^\s+//; $string =~ s/\s+$//; return $string; }